Imagine you’re a business owner juggling multiple Excel sheets to track sales, inventory, and customer data. One day, a critical report is needed, but the data is scattered across different files, leading to errors and delays. Sound familiar? This is where MySQL comes in, offering a centralized, scalable, and secure solution to manage your data.
However, getting all that Excel data into MySQL can seem daunting, especially if you’re not a database expert. But fear not—this article will guide you through six simple methods to import Excel into MySQL, making your data management more efficient and reliable. Whether you’re a beginner or a pro, these techniques will help you unlock the full potential of MySQL and streamline your operations.
How to Import Excel Data Into MySQL
This section will explore six different methods of importing excel data in MySQL.
Method | Requirements |
---|---|
Using LOAD DATA INFILE Command | CSV conversion required, Basic SQL knowledge |
Using MySQL Workbench | MySQL Workbench installed |
Using a Programming Language (Python) | Coding knowledge (Python/Java) |
Using Cloud Connectors | Cloud service setup (AWS/Zapier) |
Using PhpMyAdmin | CSV conversion required, PhpMyAdmin access |
Using Sqlizer.io | Sqlizer login |
Before diving into the methods, ensure you have the following:
- MySQL installed and running on your machine (MySQL installation guide).
- Access to MySQL Workbench or a similar client.
- Basic understanding of SQL.
We'll use a simple example file named employee_data.xlsx
, which contains the following data to explain all the methods:
Employee ID | Name | Department | Salary |
---|---|---|---|
001 | John Doe | Sales | 50,000 |
002 | Jane Smith | Marketing | 55,000 |
003 | Emily Davis | IT | 60,000 |
004 | Michael Brown | HR | 48,000 |
Method 1: Using LOAD DATE INFILE
One of the most efficient methods is to use the LOAD DATA statement. In this method, we first need to convert the Excel file into a CSV format and then use the LOAD DATA statement to import it into a MySQL table.
Step 1: Convert the Excel file into a CSV format
First, convert employee_data.xlsx
to CSV format using Excel or any other spreadsheet tool.
Step 2: Open MySQL Workbench and connect to the MySQL server
After converting the Excel file into CSV format, open the MySQL Workbench and connect to the MySQL server where you want to import the data.
Step 3: Create the employees table in your MySQL database:
Define the table name, specify the column names, and assign the appropriate data types for each column where your data will be stored.
CREATE TABLE employees (
employee_id VARCHAR(10),
name VARCHAR(50),
department VARCHAR(50),
salary DECIMAL(10,2)
);
Step 4: Enter the LOAD DATA statement
Enter the LOAD DATA statement in the MySQL Workbench to import the CSV file into a MySQL table. The LOAD DATA statement syntax is as follows:
LOAD DATA INFILE '/path/to/employee_data.csv'
INTO TABLE employees
FIELDS TERMINATED BY ','
LINES TERMINATED BY '\n'
IGNORE 1 ROWS; -- To skip the header row
In this statement, we specify the path and file name of the CSV file, the table name where we want to import the data, the field separator, the text delimiter, and the line terminator. We also use the IGNORE keyword to skip the header row of the CSV file.
Method 2: Using MySQL Workbench
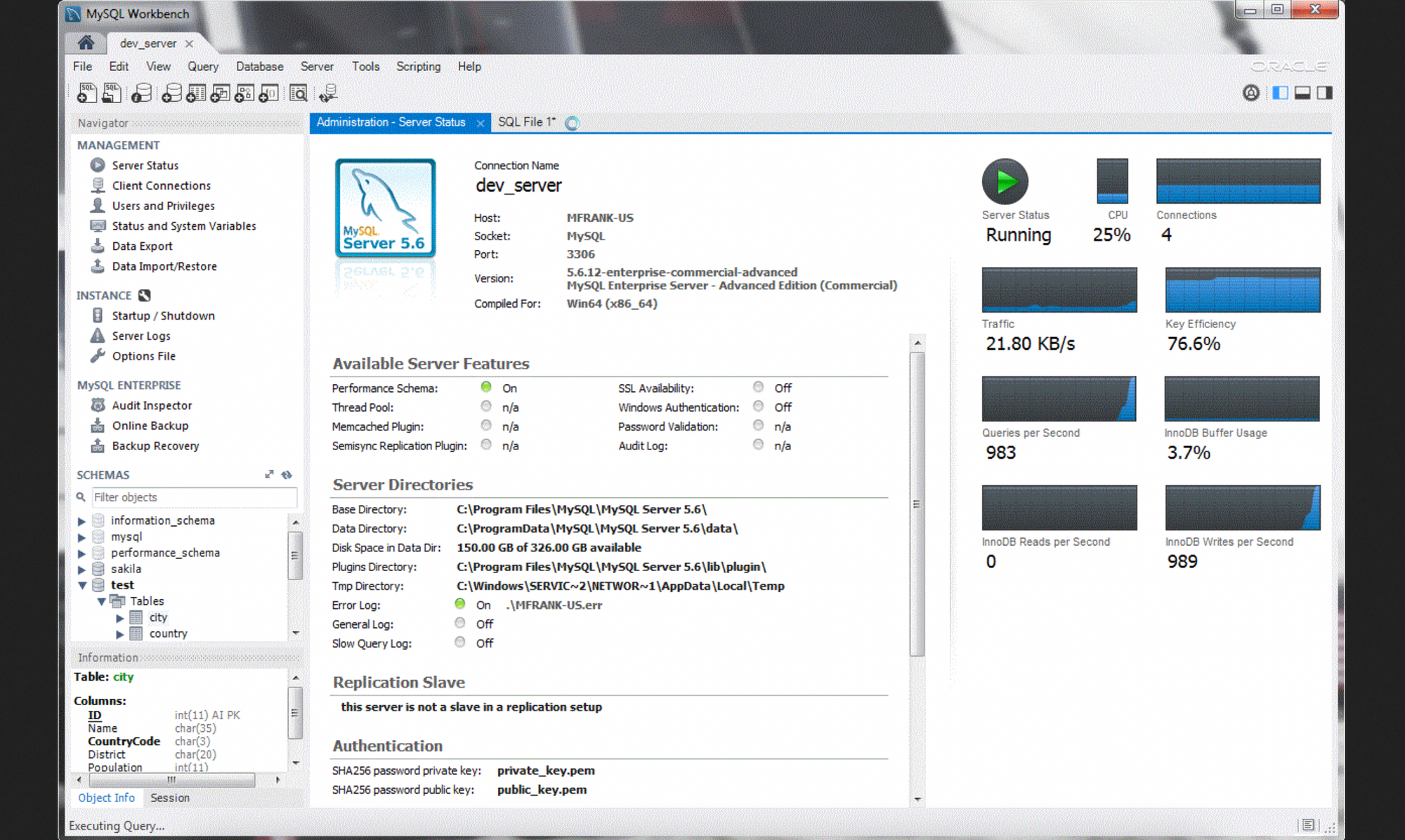
MySQL Workbench is a popular tool for managing MySQL databases and provides an easy-to-use interface for importing data from various sources, including Excel. Here’s how to import Excel data into MySQL using MySQL Workbench.
Step 1: Launch MySQL Workbench
Open MySQL Workbench on your computer and connect to your MySQL server using your credentials. You should see the main Workbench interface with your MySQL server listed.
Step 2: Access Data Import
In the top menu bar, click on Server. From the dropdown menu, select Data Import. This will open the Data Import wizard.
Step 3: Select Your Excel File
In the Data Import window, select Import from Self-Contained File. Click the Browse button next to the file path field. Navigate to and select your Excel file from your computer, then click Open.
Step 4: Choose the Target Database
Under Target Schema, choose the database where you want to import the data. If needed, select Create new table and enter a name for the new table that will store your data.
Step 5: Set Column Options
In Advanced Options, check the box for Use first row as column names if your Excel file has headers. This ensures that MySQL Workbench will use the first row of your Excel file as column names in the new table.
Step 6: Start the Import
Click the Start Import button to begin the process. Wait for the import to complete. A confirmation message will appear when the import is finished successfully.
To verify that the data has been imported, open the MySQL Workbench and navigate to the database where you imported the data. From there, you can view the newly created table and examine the data to ensure everything looks correct.
Method 3: Using a Programming Language (Python)
Pandas is a Python library that provides easy-to-use data structures and data analysis tools.Pandas offer powerful features for working with Excel data and integrating it into MySQL databases. This section will explore how to use Pandas to import Excel data into MySQL databases, including step-by-step instructions and code samples. So let's get into it.
Step 1: Install MySQL connector:
pip install pandas mysql-connector-python
Step 2: Load Pandas and MySQL connector Library
import pandas as pd
import mysql.connector
Step 3: Read the Excel file
df = pd.read_excel('employee_data.xlsx')
Step 4: Create MySQL connection
conn = mysql.connector.connect(
host='localhost',
user='root',
password='yourpassword',
database='yourdatabase'
)
cursor = conn.cursor()
Step 5: Create table if it does not exist already
cursor.execute("""
CREATE TABLE IF NOT EXISTS employees (
employee_id VARCHAR(10),
name VARCHAR(50),
department VARCHAR(50),
salary DECIMAL(10,2)
);
""")
Step 6: Insert data in MySQL
# Insert DataFrame to MySQL
for i, row in df.iterrows():
sql = "INSERT INTO employees (employee_id, name, department, salary) VALUES (%s, %s, %s, %s)"
cursor.execute(sql, tuple(row))
conn.commit()
cursor.close()
conn.close()
Download Full Code:
Method 4: Using Cloud Connectors
Cloud connectors are a convenient and powerful manner through which MySQL databases can be integrated with Excel, empowering users to analyze, visualize, and manipulate their data using the powerful features in Excel. Here, we detail how cloud connectors work between MySQL and Excel, some of their benefits, key considerations, and an essential setup guide.
Step 1: Choose a Cloud Connector:
The available types and options of the cloud connectors are diverse, ranging from Microsoft Power Query for Excel and Zapier to third-party connectors like Skyvia, CData or Devart Excel Add-ins.
Step 2: Installation and Configuration:
First, download the connector software or add-in and install it on your system. The first time you use it, configure it to connect with your MySQL database. This will usually be through your database URL, username, and password.
Step 3: Import Data into Excel:
- Open Excel and go to the Data tab.
- Click 'Get Data' (or the corresponding operation according to the connector).
- Choose your source of MySQL database.
- Use the Wizard provided to select those tables and fields you want to import into Excel.
Step 4: Data Manipulation:
Carry out data manipulation using different data analysis and data manipulation tools in Excel to generate necessary reports or dashboards and even perform statistical analysis.
Step 5: Automation and Scheduling:
Several of these connectors can be used to facilitate automatic data refreshing. Schedule for your data to be updated automatically at regular intervals in Excel so that every time you access the information, it remains current.
Step 6: Security:
Setup security measures to protect your data. This may include configuring secure connections, using VPNs, and setting up proper permissions for the right people to be able to access data in Excel.
Cloud connectors from MySQL to Excel are a way of increasing data handling capacity, specifically when there is a requirement for large datasets for complex calculations and decisions. Choose your cloud connector correctly and make the correct configuration settings to experience an increased data workflow with improved operational efficiency.
Method 5: Export MySQL Data to Excel Using PHPMyAdmin
PHPMyAdmin is free and open-source software that acts as an administration tool for both MySQL and MariaDB. It's a web-based application and is used in administering databases, tables, columns, relationships, indexes, users, permissions, and much more from any browser.
You can effectively manage and export your data out of MySQL into Excel using PHPMyAdmin with the following simple steps:
Step 1: Log into PHPMyAdmin
Start by logging into your PHPMyAdmin panel using your web address, username, and password.
Step 2: Select Your Database
From the dashboard, choose the database that contains the data you want to export. The list of databases is on the left-hand side.
Step 3: Choose Your Table
Once you've selected the database, click on the table you wish to export. This will open the table's data and options.
Step 4: Export Data
Navigate to the Export tab. Choose Quick for a straightforward export or Custom if you need more control. Select "CSV for MS Excel" as the format.
Step 5: Download the File
Click Go to generate and download your CSV file. It will be saved to your computer for easy access.
Step 6: Open and Analyze in Excel
Open Excel, go to the Data tab, and select From Text/CSV. Import your file, then use Excel’s tools to analyze and visualize your data.
Method 6: Using Sqlizer.io
Follow these steps to convert the data that you have currently in the Excel using Sqlizer.io:
Step 1: Prepare Your Excel File
Ensure your Excel file is well-formatted with clear row and column headings. Remove any blank rows or columns to avoid errors during conversion.
Step 2: Visit Sqlizer.io
Navigate to Sqlizer.io in your web browser.
Step 3: Upload Your Excel File
Click the Browse button to select and upload your Excel file. Make sure Excel Spreadsheet (.xlsx) is selected as the file type. If your first row contains column names, check the Has Header Row box.
Step 4: Configure Conversion Settings
Enter the worksheet name (e.g., Excel_to_mySQL). If needed, specify a cell range (e.g., A1:D5), and set the database table name (e.g., employee_data).
Step 5: Convert to SQL
Click Convert My File to generate the SQL script from your Excel data.
Step 6: Download and Import SQL Script
Download the SQL script file. You can import it into MySQL using MySQL Workbench by opening a new SQL tab and executing the script, or via Command Line using:
mysql -u username -p database < script.sql
Step 7: Import SQL Script into MySQL:
In your MySQL Workbench Open a new SQL tab, load the SQL script, and execute it.
Common Issues You May Encounter and How To Fix Them
Sometimes, you can follow the steps precisely and still experience errors. Let’s explore some common pitfalls and how to tackle them so you can smoothly get your data where it needs to be.
Issue | Solution |
---|---|
File format issues | Save your Excel file as CSV or XLSX. If in another format, convert it using Excel or an online tool. |
Data type issues | Ensure data types in Excel match those in MySQL tables. Modify Excel data or MySQL schema if needed. |
Encoding issues | Ensure encoding in Excel matches MySQL's (e.g., UTF-8). Convert encoding using Excel or a text editor. |
Syntax errors | Verify syntax in LOAD DATA statements or SQL queries. Use proper formatting and consult documentation. |
Expert Tips for Importing Excel Data into MySQL
Importing data from Excel into MySQL can be a complex and time-consuming process, but it doesn’t have to be. With the right tools and techniques, you can import your data efficiency and accurately while avoiding common pitfalls. With this in mind, here are some expert tips for importing your excel data into MySQL.
- Clean Your Data First: Before importing, make sure your Excel data is clean and properly formatted. This means removing unnecessary formatting, ensuring consistency, and checking for blank or null values. Clean data minimizes import errors and saves you time in the long run.
- Use Unique Identifiers: Assign a unique identifier to each record. This not only keeps your data accurate and consistent but also makes searching and updating records much easier.
- Batch Insert for Speed: For large datasets, use batch inserts. By breaking the data into smaller chunks, you can speed up the import process and reduce the strain on your system.
- Automate with Scripts or Tools: Instead of importing data manually, consider using a script or tool. Automation reduces the risk of errors and saves time.
- Test After Import: Once your data is in MySQL, test it. Verify that all fields are populated correctly, check for any errors or inconsistencies, and make necessary updates. This final step ensures your data is ready to use.
Import Data to SQL Using Nanonets
We rarely receive our data neatly packaged in Excel files. More often, it arrives through emails, PDF files, or even physical documents. Before we can centralize this information in a common SQL server for easy access and querying, we first need to extract the relevant data from these documents. That’s where Nanonets steps in, offering a complete end-to-end automation solution.
Imagine you receive data as PDFs uploaded to a folder in Google Drive. Nanonets can automatically detect any new file in that folder, extract the necessary information, and seamlessly import it into your SQL server. Let’s walk through how you can set this up step by step.
Step 1: Sign In to Nanonets and Create a New Workflow
Begin by signing in to Nanonets, which you can do for free. Simply visit Nanonets and click on "Get Started for Free." Once you're in, navigate to the "Create your own workflow" option.
Step 2: Set Up File Origination and Destination
Next, decide where your PDF files are stored and where you want the extracted data to be transferred. This involves selecting the source platform—Google Drive, for instance—and the destination, which in this case is your PostgreSQL database. This setup ensures that Nanonets knows exactly where to pull the files from and where to send the processed data.
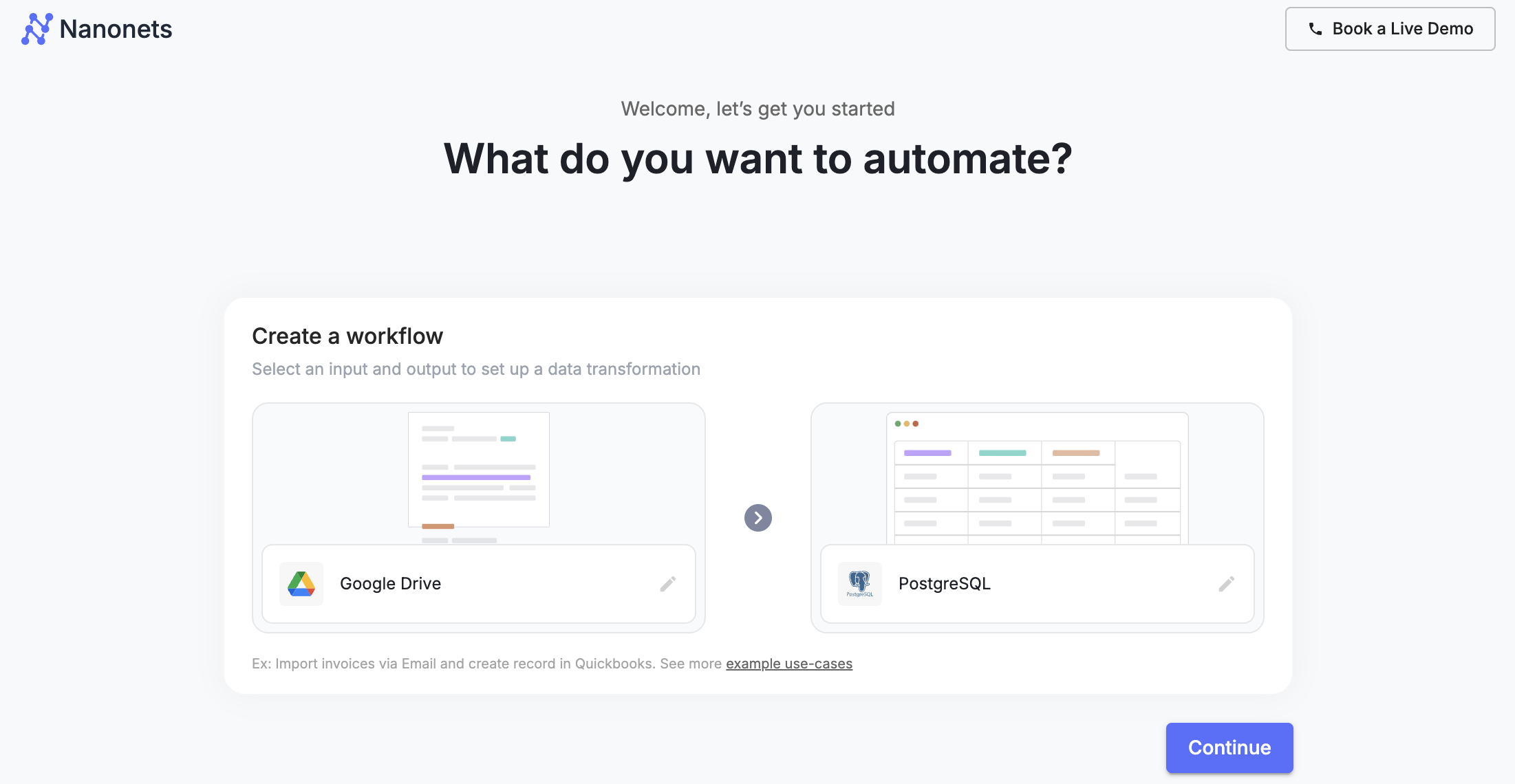
Step 3: Connect Your Account and Choose File Location
Now, connect your Google Drive account to Nanonets. This connection allows Nanonets to access your files directly from your cloud storage. After linking your account, browse through your Google Drive folders and select the specific folder where your PDF files are stored.
Step 4: Nanonets Extracts Data from the Document
Here’s where the magic happens! Nanonets GenAI will automatically extract data from your PDF files. The platform uses advanced AI to accurately capture and organize the information from your documents. Before finalizing, Nanonets will display the extracted data for you to cross-check and approve. This validation step ensures that the data is accurate before it’s transferred to your database.
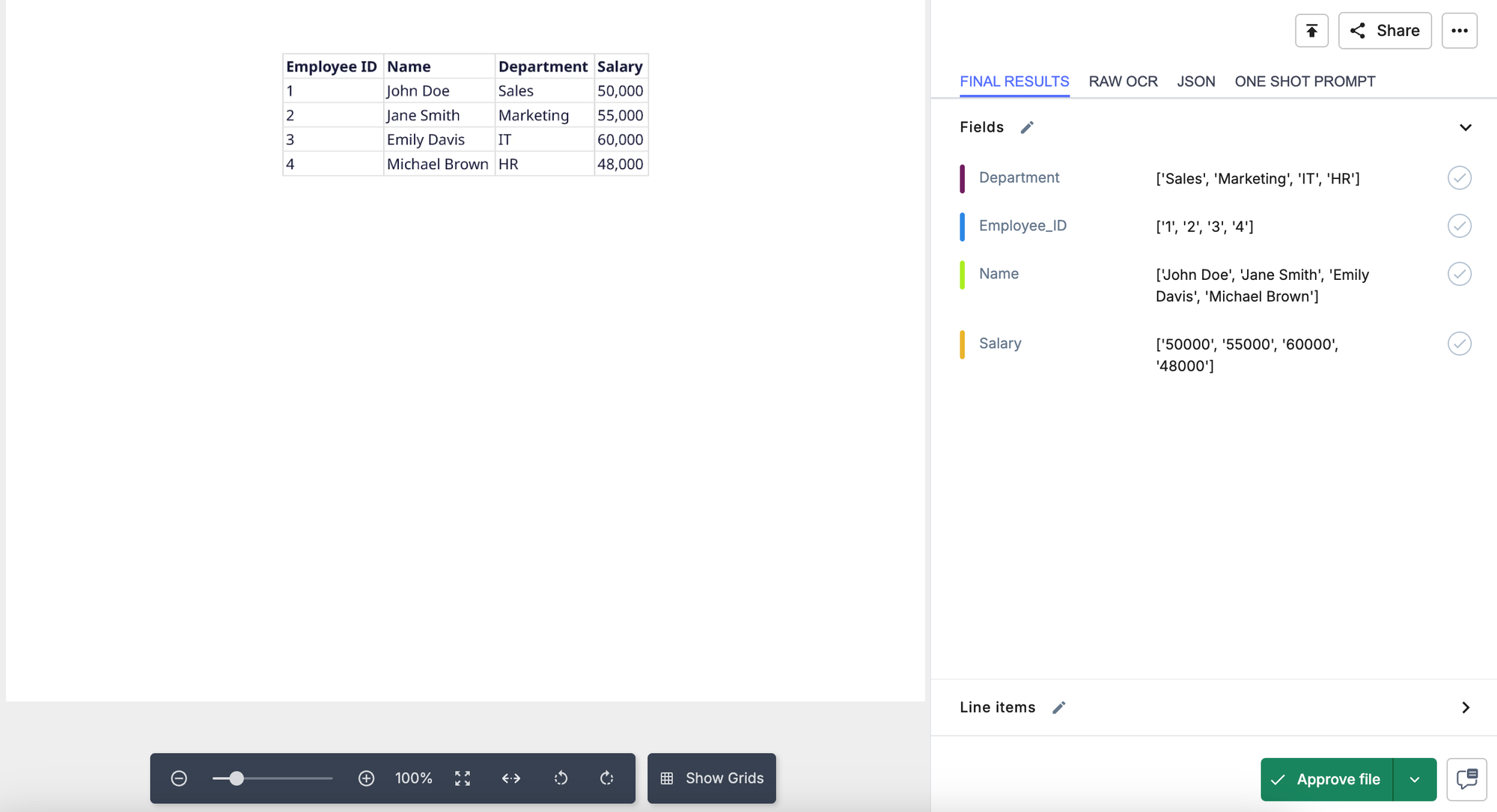
Step 5: Select the Data and Table
After approving the extracted data, it’s time to select where this data will be stored. Choose the specific database and table within PostgreSQL where the data should be saved.
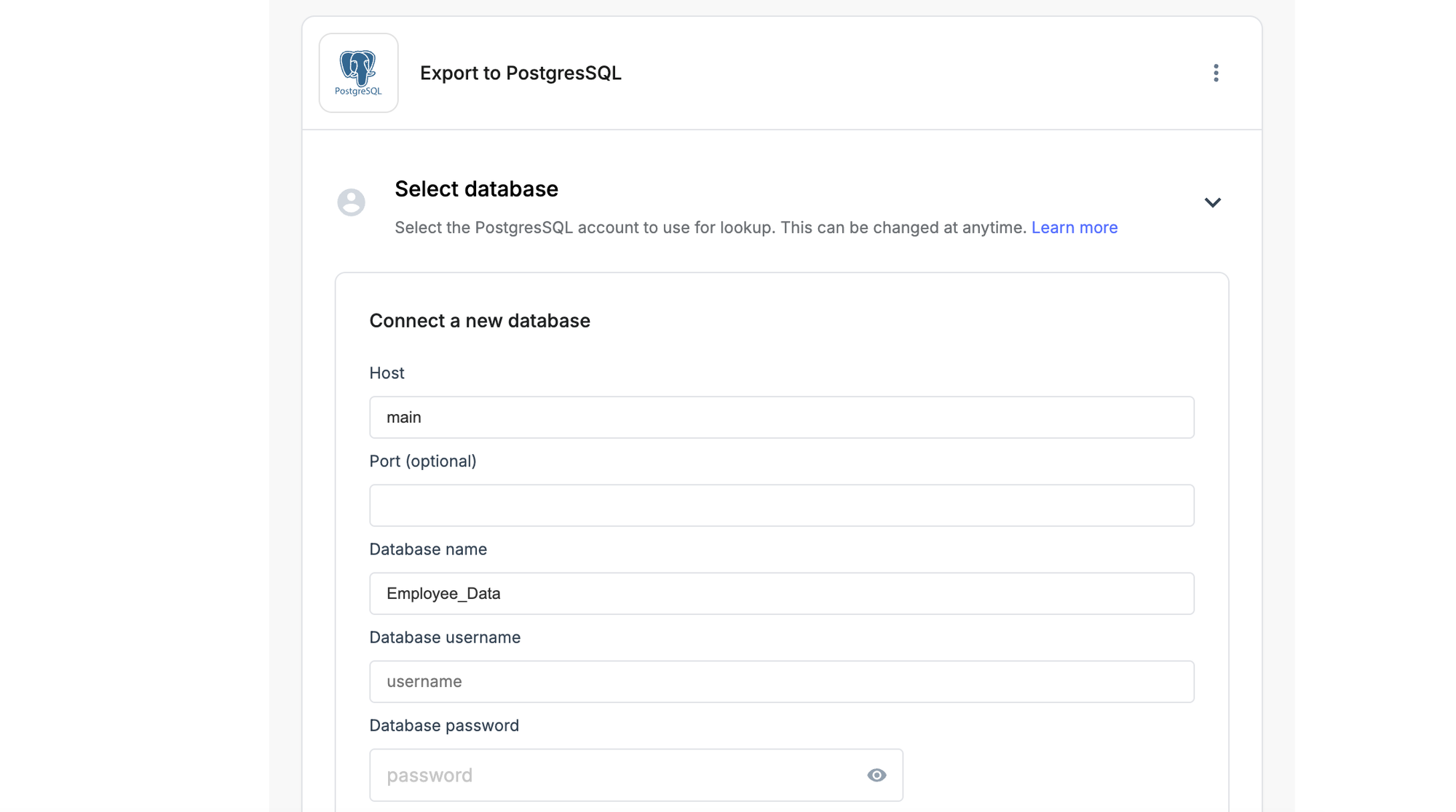
Step 6: Map Nanonets Columns to Table Columns
To complete the setup, you need to map the columns extracted by Nanonets to the corresponding columns in your PostgreSQL table. This mapping process is straightforward, thanks to Nanonets’ intuitive interface. By aligning the data fields correctly, you ensure that the imported data integrates seamlessly into your database structure.
All Done!
Once the setup is complete, Nanonets takes over. Any new PDF file added to the selected Google Drive folder will be automatically processed, with the data extracted and transferred to your PostgreSQL server. This automation saves you time, reduces the risk of errors, and ensures that your database is always up-to-date with the latest information.
By following these steps, you can efficiently manage your data imports, leveraging the power of Nanonets to handle the heavy lifting. Whether you're dealing with a few documents or large volumes of data, Nanonets makes the process smooth, reliable, and scalable.
Nanonets can automate data entry into MySQL. Have a use case in mind? Start a free trial or reach out to our team.
Conclusion
By integrating Excel data into MySQL, you gain centralized storage, robust security, and powerful querying capabilities. Adopting MySQL improves scalability and data integrity, driving better business decisions and productivity. Implement these methods today to unlock the full potential of your data. For more tips and detailed guides on efficient data management, explore our blog and stay ahead in the data games!
FAQs
Can I import multiple Excel sheets into a single MySQL table?
Yes, most methods allow you to import multiple sheets by specifying the sheet name or consolidating them into a single CSV file before import.