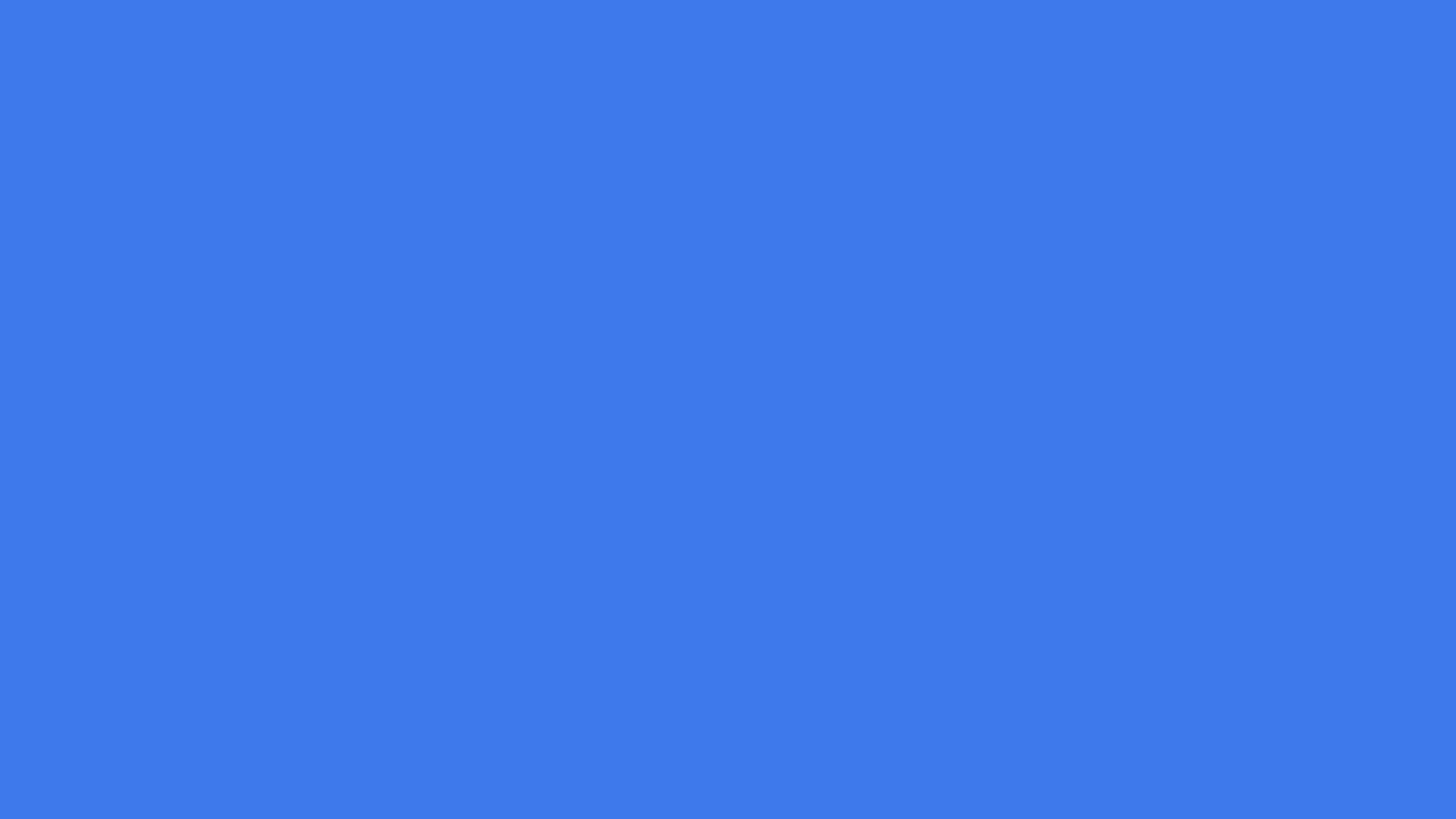
All businesses in the world deal with documents. These documents can be physical or digital but they are usually scattered across filing cabinets, or digital storages, like email inboxes or cloud storages. This essentially means valuable structured insights squandered in this mess.
In this age of artificial intelligence, there are technologies that solve for this. Cutting-edge technologies, like Optical Character Recognition (OCR) and Natural Language Processing (NLP) make it easy to extract structured information from these documents and turn them into valuable insights.
Google Document AI provides the underlying technology along with a suite of self-serve machine learning tools for anyone to create document extractors. In this article, we will explore what Google document AI is, how to get started with it, the technologies and the applications of Google document AI and also look at alternatives to Google document AI that specialize in data extraction from documents.
What is Google document AI?
Put simply, Google document AI provides the underlying technology you need to create artificially intelligent document extractors. This can help you turn unstructured data into structured information that can help unlock strategic, actionable insights.
Think documents like invoices, receipts, contracts, forms, etc. scattered across cloud storages, email inboxes, etc. processed and all relevant details captured into a structured database, automatically. This process is free from manual errors, is fast and efficient and increases the accessibility of data multifold.
For the more nuanced audiences, Google document AI is a suite of machine learning tools designed to automate the extraction and processing of information from documents. Powered by Google’s state-of-the-art AI technologies such as, natural language processing (NLP) and optical character recognition (OCR), it allows businesses to turn unstructured data—such as invoices, receipts, contracts, and forms—into structured, actionable insights.
How does Google Document AI work?
Google document AI, can be thought of as an amalgamation of several Artificial Intelligence technologies. While this is not black and white, there are two important ones that stand out. Computer Vision, powered by CNNs, as the name suggests, equips machines with vision. Simply put, it enables machines to interpret visual data. The second one is, Natural Language Processing (NLP), specifically Optical Character Recognition (OCR) which enables machines to identify text within PDFs and understand the significance and correlation between that text as a human would perceive.
Convolutional Neural Networks (CNNs)
With the advent of deep learning, traditional methods for extracting features from images have been surpassed due to their lower accuracy. Modern image analysis largely relies on convolutional neural networks (CNNs).
CNNs are specialised neural networks that use convolutional layers to process images. Unlike earlier methods where the parameters of convolutional filters were fixed, CNNs learn these parameters through training. This learning capability enables CNNs to identify complex features in images, such as text, which traditional methods struggled with due to their rigid, predefined filters.
Interestingly, while the concept of CNNs has been around for decades, their practical use only became feasible recently due to advances in computational power. Today, CNNs are at the heart of advanced vision tasks, including object recognition, segmentation, and more.
In essence, CNNs in Google Document AI are used to detect text, key-value pairs, and tables from PDFs.
Natural language processing (NLP)
Similar to the advancements in image analysis, deep learning has revolutionised the field of language interpretation, or NLP. NLP involves understanding and deriving meaning from text, which can be more complex than image interpretation due to the nuances of language.
Recent developments in NLP have focused on long-short-term memory (LSTM) networks, which analyse sequences of words by considering both the current and past context. More recently, the spotlight has shifted to transformers, a type of network that excels at capturing the importance of different words in a sentence relative to each other. Transformers have significantly improved performance in various NLP tasks, including semantic understanding and contextual word navigation.
Getting Started with Google Document AI
Now that we understand the technologies that go behind Google Document AI, let’s take a look at basic code scripts that can help you leverage Google document AI for document management and data extraction.
To get started with Google Document AI, you'll need to meet several general prerequisites:
- Install client libraries for your preferred programming language (e.g., Python, Java) to interact with Document AI APIs. These libraries simplify the process of making API requests.
- Create and configure a service account if you need programmatic access. This involves generating and downloading a JSON key file for authentication.
- Ensure that you have the necessary Identity and Access Management (IAM) permissions. Typically, you'll need roles such as roles/documentai.admin or roles/documentai.user depending on your access needs.
- Enable the Document AI API for your Google Cloud project. This can be done through the Google Cloud Console under the API & Services section.
- Link a billing account to your Google Cloud project. Document AI is a paid service, and having a billing account ensures you can access and use the service.
- Set up a Google Cloud project through the Google Cloud Console. This project will act as the container for all your resources and services.
- Create a Google Cloud account if you don't already have one. This account will give you access to Google Cloud services and billing.
Now that you have the basics squared away, we are ready to explore how we can set up basic document management functions, like, extracting plain text from documents, extracting entities, relationships and sentiments from text as a human would as well as classifying a few documents into arbitrarily-defined categories. Let’s dive in.
1. Data Extraction using Optical Character Recognition (OCR)
Optical Character Recognition (OCR) is a critical technology in Document AI, enabling the extraction of text from scanned documents and images. Google Document AI uses advanced OCR techniques to recognise and digitise text from various document formats, making it searchable and editable.
This is best suited for extracting static data points that appear on the documents themselves, for instance, invoice number and amount due from an Invoice, driver name and nationality from a driver’s license, etc.
To perform OCR using Google Cloud Vision API, you can use the following Python code snippet:
from google.cloud import vision_v1
from google.cloud.vision_v1 import types
def detect_text(path):
client = vision_v1.ImageAnnotatorClient()
with open(path, 'rb') as image_file:
content = image_file.read()
image = types.Image(content=content)
response = client.text_detection(image=image)
texts = response.text_annotations
for text in texts:
print('\n"{}"'.format(text.description))
if __name__ == "__main__":
detect_text('path/to/your/document.jpg')
This script uses Google’s Vision API to extract text from an image file, providing a foundation for further processing.
2. Entity, Relationship and Sentiment Analysis using Natural Language Processing (NLP)
Natural language processing (NLP) helps Google Document AI understand and analyze human language within documents. It enables the system to extract meaningful information, such as entities, sentiment, and relationships from text.
This is best suited for sentiment analysis, relationship analysis, named entity recognition etc. between data points contained in the document. This API within Google Document AI allows you to analyse the subtext as perceived by a human from within the text.
Here’s an example of how to recognise named entities using the Google Cloud Natural Language API:
from google.cloud import language_v1
def analyze_entities(text):
client = language_v1.LanguageServiceClient()
document = language_v1.Document(content=text, type_=language_v1.Document.Type.PLAIN_TEXT)
response = client.analyze_entities(document=document)
for entity in response.entities:
print('Entity: {}'.format(entity.name))
print('Type: {}'.format(language_v1.Entity.Type(entity.type_).name))
print('Salience: {}'.format(entity.salience))
if __name__ == "__main__":
analyze_entities("Google Document AI is an advanced tool for document processing.")
This code snippet uses the Natural Language API to analyze entities in a given text, highlighting the entities and their types.
3. Document Classification using AutoML
AutoML is another powerful feature of Google Document AI. It allows users to train custom machine learning models tailored to their specific document types and processing needs. This customisation can enhance the accuracy and relevance of data extraction.
This is best suited for document or image classification.
To use AutoML with Document AI for document classification, you typically need to perform several steps, including setting up the Google Cloud environment, creating a dataset, training a model, and deploying it.
from google.cloud import documentai_v1beta3 as documentai
from google.cloud import aiplatform
# Set up the environment
project_id = 'your-project-id'
location = 'us' # or your specific region
dataset_id = 'your-dataset-id'
model_id = 'your-model-id'
document_id = 'your-document-id'
# Initialize Document AI client
client = documentai.DocumentUnderstandingServiceClient()
# Initialize AutoML client
aiplatform.init(project=project_id, location=location)
# Load document for classification
def load_document(document_id):
# This is a placeholder function. You should implement the actual method to load or create a Document object.
return {
'name': f'projects/{project_id}/locations/{location}/documents/{document_id}'
}
# Predict using the AutoML model
def classify_document(document_id):
# Load the document
document = load_document(document_id)
# Get the AutoML model
model = aiplatform.Model(model_id)
# Predict
response = model.predict([document])
# Display results
print("Prediction results:")
for prediction in response.predictions:
print(prediction)
if __name__ == '__main__':
classify_document(document_id)
Applications of Google Document AI
Google Document AI has a broad range of applications across various industries. Here are some specific use-cases:
1. Invoice Processing
Automating invoice processing is a primary use-case for Google Document AI. It can extract key information from invoices, such as amounts, dates, and vendor details, reducing manual data entry and improving accuracy.
Use Case Example: A company receives hundreds of invoices monthly. Using Google Document AI, the company can automatically extract invoice details, validate them against purchase orders, and input them into accounting systems, saving significant time and reducing errors.
2. Forms and Surveys
Google Document AI can process forms and survey responses by extracting data from filled forms and consolidating responses for analysis.
Use Case Example: An organization conducting customer satisfaction surveys can use Document AI to process the responses from scanned forms, automatically extracting and organising feedback data for further analysis.
3. Contract Management
In contract management, Google Document AI helps in extracting key terms, clauses, and conditions from contracts. This assists in contract review, compliance checks, and managing renewal dates.
Use Case Example: A legal department uses Document AI to extract renewal dates and important clauses from a portfolio of contracts, enabling better contract management and compliance monitoring.
4. Medical Records
In healthcare, Google Document AI can assist in digitising and extracting information from medical records, facilitating easier access and integration into electronic health records (EHR) systems.
Use Case Example: A hospital uses Document AI to convert paper-based patient records into digital formats, extracting relevant medical history and treatment details for better patient care and record-keeping.
5. Legal Document Analysis
For legal professionals, Google Document AI aids in analysing legal documents, identifying pertinent information, and assisting in case preparation.
Use Case Example: A law firm uses Document AI to process and analyse legal briefs, automatically extracting case details, legal precedents, and critical information to streamline case research and preparation.
Alternatives to Google Document AI
Google document AI may be a powerful solution for unlocking structured information from unstructured documents. It offers a suite of machine learning tools geared towards facilitating development. In this section, we will take a look at 3 top alternatives to Google document AI that have somewhat similar offerings:
1. Amazon Textract
Amazon Textract is a cloud-based service from AWS designed to automatically extract text, forms, and tables from scanned documents and images. It combines optical character recognition (OCR) with machine learning to analyse and understand document content, making it easier for organisations to process and manage large volumes of paperwork.
Key Features:
Advanced Text Extraction: Extracts printed text and handwriting from a wide range of document types, including forms and tables.
Form and Table Extraction: Identifies and extracts structured data from forms and tables, preserving the format and relationships between data fields.
Built-In OCR: Uses sophisticated OCR technology to convert scanned images and PDFs into machine-readable text.
Integration with AWS Services: Easily integrates with other AWS services, such as Amazon S3, Lambda, and Comprehend, for seamless data processing and analysis.
Automatic Document Analysis: Leverages machine learning models to automatically detect and classify document components, improving accuracy and reducing manual intervention.
Related Read: Amazon Textract Alternatives for Data Extraction
2. Microsoft Azure Form Recogniser
Microsoft Azure Form Recogniser is an AI-powered service that extracts key information from forms, documents, and receipts. It leverages machine learning and OCR to automate data entry and document processing, providing flexible and scalable solutions for various data extraction needs.
Key Features:
Pre-built and Custom Models: Offers both pre-built models for common document types and the ability to train custom models for specialised document formats.
Text and Data Extraction: Captures text and key data from a wide variety of documents, including invoices, receipts, and business forms.
Flexible Deployment: Can be integrated with other Azure services, such as Azure Logic Apps and Power Automate, for enhanced workflow automation and data management.
Real-time Processing: Provides real-time data extraction and processing capabilities, ensuring up-to-date information for timely decision-making.
High-Volume Scalability: Designed to handle large volumes of documents efficiently, making it suitable for enterprise-level applications.
3. ABBYY FlexiCapture
ABBYY FlexiCapture is a comprehensive data capture and document processing platform that combines OCR, machine learning, and advanced data extraction technologies. It is designed to handle complex document types and workflows, providing high accuracy and flexibility for data management.
Key Features:
Multi-Channel Data Capture: Supports data extraction from various sources, including paper documents, digital images, and emails.
Advanced Document Classification: Uses machine learning to automatically classify and categorise documents, improving organization and retrieval.
Customisable Templates: Allows users to create and customise extraction templates to fit specific document layouts and data fields.
End-to-End Processing: Offers a complete data capture solution, from initial document scanning to data validation and integration with enterprise systems.
Integration Capabilities: Integrates with a wide range of enterprise applications and systems, facilitating seamless data flow and process automation.
While these alternatives provide robust features for intelligent AI-based document processing and data extraction, similar to Google Document AI, and can be integrated into various business workflows to enhance efficiency and accuracy, there are some that do not appear on this list. IBM Watson Discovery and Laserfiche happen to be popular choices as well.
There are alternatives in the market that are ready-to-use. These tools are specialised in data extraction and offer simpler, no-code user interfaces, pre-trained models catering to different document types as well as workflow automation capabilities. A few alternatives to Google document AI that specialise with data extraction and workflow automation are Nanonets, Rossum, Super.AI and a few others.
Conclusion
Google Document AI represents a significant advancement in automating document processing, leveraging powerful technologies like OCR, NLP, and machine learning. Its applications span various industries, from invoice processing to medical records. However, alternatives like Nanonets also offer robust solutions, with customisable features and user-friendly interfaces.
Choosing the right tool depends on your specific needs, the types of documents you handle, and the level of customisation required. Whether you opt for Google Document AI or explore alternatives like Nanonets, embracing these technologies can greatly enhance efficiency and accuracy in managing document-based data.